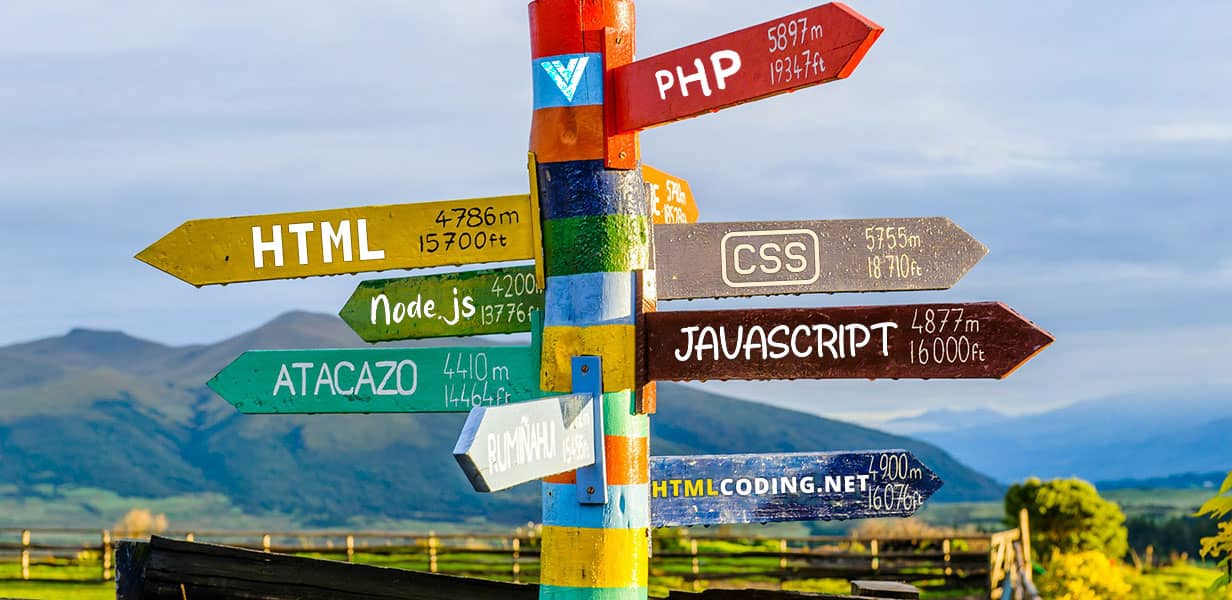
Coding Guidelines
General
Golden rules
Every line of code should look like it’s written by one person, regardless of contributors.
- Use US English for comments, classes, and variable names.
- Prioritize readability over brevity: Choose descriptive, clear names for variables and methods. CPU and memory is cheap; human hours are not.
- Avoid cryptic abbreviations (e.g.,
$pc_id
). Use$process_id
instead. - Avoid vague names like
$var
or$data
. Be specific about contents. E.g.,$user
Editor preferences
Set your editor to the following settings to avoid common code inconsistencies and dirty diffs:
- Use soft-tabs set to two spaces.
- Trim trailing white space on save.
- Set encoding to UTF-8.
- Add new line at end of files.
Consider documenting and applying these preferences to your project’s .editorconfig
file.
root = true
[*]
charset = utf-8
end_of_line = lf
indent_size = 2
indent_style = space
insert_final_newline = true
trim_trailing_whitespace = true
PUG/HTML
Validation
All HTML pages should be verified against the W3C validator to ensure that the markup is well formed.
Syntax
- Don’t capitalize tags, including the doctype.
- Use soft tabs with two spaces—they’re the only way to guarantee code renders the same in any environment.
- Nested elements should be indented once (two spaces).
- Always use double quotes, never single quotes, on attributes.
- Don’t include a trailing slash in self-closing elements.
- Don’t omit optional closing tags (e.g.
</li>
or</body>
).
Example:
<!doctype html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1 class="sec-title">Hello, world!</h1>
<img class="sec-banner" src="img/common/logo.png" alt="Company">
</body>
</html>
Define Charset
The W3C always recommend developers to declare the charset or character encoding explicitly. It can be done by using the charset attribute of <meta>
tag. Pass utf-8
as a value to charset attribute as it is the most commonly used character encoding and provides over a million characters.
<meta charset="utf-8" />
Set the viewport
Setting the viewport helps web pages render well on different devices. It is achieved by controlling the width and scale of the page. It is used for ensuring the responsiveness of a particular web page.
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
IE compatibility mode
There’s no need to include the Internet Explorer document compatibility <meta>
tag these days, unless you need support for IE10 and older.
<!-- IE10 and below only -->
<meta http-equiv="x-ua-compatible" content="ie=edge">
CSS and JS includes
No need to specify a type when including CSS and JS files as text/css
and text/javascript
are their respective defaults.
<!-- External CSS -->
<link rel="stylesheet" href="styles.css">
<!-- In-document CSS -->
<style>
/* ... */
</style>
<!-- JS -->
<script src="scripts.js"></script>
Boolean attributes
A boolean attribute is one that needs no declared value.
<input type="text" disabled>
<input type="checkbox" value="1" checked>
<select>
<option value="1" selected>1</option>
</select>
Attributes and Tags
All tags and attributes must be written in lowercase. Additionally, attribute values should be lowercase when the purpose of the text therein is only to be interpreted by machines. For instances in which the data needs to be human readable, proper title capitalization should be followed.
For machines:
<meta http-equiv="content-type" content="text/html; charset=utf-8" />
For humans:
<a href="http://example.com/" title="Description Here">Example.com</a>
Quotes
All attributes must have a value, and must use double-quotes. The following are examples of proper and improper usage of quotes and attribute/value pairs.
Correct:
<input type="text" name="email" />
Incorrect:
<input type=text name=email>
SCSS/CSS
Structure
There are plenty of different methods for structuring a stylesheet. With the CSS in core, it is important to retain a high degree of legibility. This enables subsequent contributors to have a clear understanding of the flow of the document.
- Use 2 spaces, not tabs, to indent each property.
- Add two blank lines between sections and one blank line between blocks in a section.
- Each selector should be on its own line, ending in either a comma or an opening curly brace. Property-value pairs should be on their own line, with one tab of indentation and an ending semicolon. The closing brace should be flush left, using the same level of indentation as the opening selector.
Correct:
.selector-1,
.selector-2,
.selector-3 {
background: #fff;
color: #000;
}
Incorrect:
.selector-1, .selector-2, .selector-3 {
background: #fff;
color: #000;
}
.selector-1 { background: #fff; color: #000; }
Selectors
With specificity, comes great responsibility. Broad selectors allow us to be efficient, yet can have adverse consequences if not tested. Location-specific selectors can save us time, but will quickly lead to a cluttered stylesheet. Exercise your best judgement to create selectors that find the right balance between contributing to the overall style and layout of the DOM.
- Use lowercase and separate words with hyphens when naming selectors. Avoid camelcase and underscores.
- Use human readable selectors that describe what element(s) they style.
- Attribute selectors should use double quotes around values.
- Refrain from using over-qualified selectors,
div.container
can simply be stated as.container
.
Correct:
.comment-form {
margin: 1em 0;
}
input[type="text"] {
line-height: 1.1;
}
Incorrect:
.commentForm { /* Avoid camelcase. */
margin: 0;
}
.comment_form { /* Avoid underscores. */
margin: 0;
}
div.comment_form { /* Avoid over-qualification. */
margin: 0;
}
.c1-xr { /* What is a c1-xr?! Use a better name. */
margin: 0;
}
input[type=text] { /* Should be [type="text"] */
line-height: 1.5;
}
Properties
Similar to selectors, properties that are too specific will hinder the flexibility of the design. Less is more. Make sure you are not repeating styling or introducing fixed dimensions (when a fluid solution is more acceptable).
- Properties should be followed by a colon and a space.
- All properties and values should be lowercase, except for font names and vendor-specific properties.
- Use hex code for colors, or rgba() if opacity is needed. Avoid RGB format and uppercase, and shorten values when possible:
#fff
instead of#FFFFFF
. - Use shorthand, except when overriding styles, for
background
,border
,font
,list-style
,margin
, andpadding
values as much as possible. For a shorthand reference, see CSS Shorthand.
Correct:
.selector {
background: #fff;
display: block;
margin: 0;
margin-left: 20px;
}
Incorrect:
.selector {
background: #FFFFFF;
display: BLOCK;
margin-left: 20PX;
margin: 0;
}
Property Ordering
Group like properties together, the baseline for ordering is:
- Display
- Positioning
- Box model
- Colors and Typography
- Other
Vendor Prefixes
We use Autoprefixer in our Gulp Task Runner to easily manage necessary browser prefixes. For works without using our Gulp, vendor prefixes should go longest (-webkit-) to shortest (unprefixed). All other spacing remains as per the rest of the standards.
.sample-output {
-webkit-box-shadow: inset 0 0 1px 1px #eee;
-moz-box-shadow: inset 0 0 1px 1px #eee;
box-shadow: inset 0 0 1px 1px #eee;
}
Values
There are numerous ways to input values for properties. Follow the guidelines below to help us retain a high degree of consistency.
- Space before the value, after the colon.
- Do not pad parentheses with spaces.
- Always end in a semicolon.
- Use double quotes rather than single quotes, and only when needed, such as when a font name has a space or for the values of the
content
property. - Font weights should be defined using numeric values (e.g.
400
instead ofnormal
,700
rather thanbold
). - 0 values should not have units unless necessary, such as with
transition-duration
. - Line height should also be unit-less, unless necessary to be defined as a specific pixel value.
- Use a leading zero for decimal values, including in
rgba()
. - Multiple comma-separated values for one property should be separated by either a space or a newline. For better readability newlines should be used for lengthier multi-part values such as those for shorthand properties like
box-shadow
andtext-shadow
, including before the first value. Values should then be indented one level in from the property. - Lists of values within a value, like within
rgba()
, should be separated by a space.
Correct:
.class { /* Correct usage of quotes */
background-image: url(images/bg.png);
font-family: "Helvetica Neue", sans-serif;
font-weight: 700;
}
.class { /* Correct usage of zero values */
font-family: Georgia, serif;
line-height: 1.4;
text-shadow:
0 -1px 0 rgba(0, 0, 0, 0.5),
0 1px 0 #fff;
}
.class { /* Correct usage of short and lengthier multi-part values */
font-family: Consolas, Monaco, monospace;
transition-property: opacity, background, color;
box-shadow:
0 0 0 1px #5b9dd9,
0 0 2px 1px rgba(30, 140, 190, 0.8);
}
Incorrect:
.class { /* Avoid missing space and semicolon */
background:#fff
}
.class { /* Avoid adding a unit on a zero value */
margin: 0px 0px 20px 0px;
}
.class {
font-family: Times New Roman, serif; /* Quote font names when required */
font-weight: bold; /* Avoid named font weights */
line-height: 1.4em; /* Avoid adding a unit for line height */
}
.class { /* Incorrect usage of multi-part values */
text-shadow: 0 1px 0 rgba(0, 0, 0, 0.5),
0 1px 0 #fff;
box-shadow: 0 1px 0 rgba(0, 0,
0, 0.5),
0 1px 0 rgba(0,0,0,0.5);
}
Media Queries
Media queries allow us to gracefully degrade the DOM for different screen sizes. If you are adding any, be sure to test above and below the break-point you are targeting.
- Place media queries as close to their relevant rule sets whenever possible.
- Don’t bundle them all in a separate stylesheet or at the end of the document. Doing so only makes it easier for folks to miss them in the future.
Example:
.element { ... }
.element-avatar { ... }
.element-selected { ... }
@media (min-width: 480px) {
.element { ... }
.element-avatar { ... }
.element-selected { ... }
}
!important
Avoid using !important
as much as possible, only if used sparingly and proactively.
Proactive use of !important
is when it is used before you’ve encountered any specificity problems; when it is used as a guarantee rather than as a fix. For example:
.is-hidden {
display: none !important;
}
JavaScript
Indentation
Use an indent of 2 spaces for each nesting level to ensure clear, compact readability. Avoid tabs, as they render inconsistently across editors and disrupt visual alignment.
Names
Functions and variables should be named using lowerCamelCase.
// Variable names in lowerCamelCase
let userName = 'Alice';
let totalPrice = 49.99;
let isLoggedIn = true;
// Function names in lowerCamelCase
function calculateDiscount(price, percentage) {
let discountAmount = price * (percentage / 100);
return discountAmount;
}
function fetchUserData(userId) {
let apiUrl = `https://api.example.com/users/${userId}`;
return fetch(apiUrl);
}
function updateStatusMessage(newStatus) {
let statusText = `Status: ${newStatus}`;
console.log(statusText);
}
Braces
Always use curly braces, even in situations where they are technically optional. Having them increases readability and decreases the likelihood of logic errors being introduced as the codebase continues to evolve.
- Opening curly braces should never be on their own new line.
- Closing curly braces should always be on their own new line.
Example:
function calculateTotal(price, quantity) {
if (price > 0) {
let total = price * quantity;
return total;
} else {
return 0;
}
}
function logMessage(isActive) {
if (isActive) {
console.log("System is active");
}
}
Semicolons
End all statements with a semicolon, except for for
, function
, if
, switch
, try
, and while
.
// Basic variable assignments and expressions
let firstName = 'John';
let lastName = 'Doe';
let fullName = firstName + ' ' + lastName;
// Function definition (no semicolon after block)
function greetUser(name) {
let message = 'Hello, ' + name;
console.log(message);
}
// If statement (no semicolon after block)
if (fullName.length > 0) {
let greeting = 'Welcome back';
console.log(greeting);
}
// For loop (no semicolon after block)
for (let i = 0; i < 3; i++) {
let count = i + 1;
console.log('Count: ' + count);
}
Operators
All binary operators (operators that come between two values), such as +
, -
, =
, !=
, ==
, >
, &&
, ||
, etc. should have a space before and after the operator, for readability.
var string = 'Foo' + bar;
var string += 'Foo';
if ((someQty < otherQty) && (someBoolean == true)) {
doStuff();
}
Unary operators (operators that operate on only one value), such as ++
, !
, etc. should not have a space between the operator and the variable or number they are operating on.
someInt++;
if (!condition) {
action();
}
Javascript has one ternary operator (operators that operate on three values) called the conditional operator. The ternary operator should have a space on either side of the ?
and the :
.
condition ? result1 : result2;